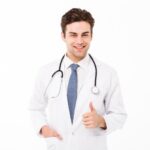
لیلا فیروزجنگ
کد نظام پزشکی : م-5128
کارشناس مامایی
تهران
تعداد 0 نظر درباره ماما لیلا فیروزجنگ وجود دارد و در کل وب سایت تعداد 2300 نظر ثبت شده است.
شما هم میتوانید نظرات را مطالعه و در صورت تمایل نظر خود را بیان کنید.
دریافت نوبت لیلا فیروزجنگ
پزشکان متخصص مشابه
در صورتی که شما کارشناس لیلا فیروزجنگ میباشید و نیاز به بروزرسانی اطلاعات این صفحه دارید ، از طریق فرم تماس با ما اطلاعات خود را ارسال تا تیم پشتیبانی سایت دکتر خوب با شما تماس گرفته و اقدامات لازم صورت پذیرد.
بیوگرافی لیلا فیروزجنگ کارشناس مامایی
لیلا فیروزجنگ کارشناس مامایی هستند. مطب لیلا فیروزجنگ در درحال بروزرسانی آدرس پزشک واقع شده است. برای مراجعه به میتوانید از پروفایل ایشان در سایت دکتر خوب به صورت اینترنتی و غیرحضوری نوبت بگیرید. همچنین در صورت نیاز به تماس با مطب می توانید با شماره تلفن درحال بروزرسانی تلفن پزشک تماس بگیرید.
تحصیلات لیلا فیروزجنگ
تحصیلات لیلا فیروزجنگ ، تخصص در رشته مامایی , است.
نظر درباره لیلا فیروزجنگ
در قسمت نظرات میتوانید از نظرات سایر مراجعین به دکتر با خبر شوید.
نزدیک ترین نوبت دکتر چه زمانی است؟
برای اطلاع از نزدیک ترین نوبت لیلا فیروزجنگ به قسمت دریافت نوبت در پروفایل ایشان مراجعه کنید. در صورتیکه دکتر نوبت خالی نداشت ، با کلیک روی گزینه « نوبت دار شد اطلاع بده » سریع تر از دیگران از اولین نوبت خالی ایشان با خبر شوید.
آدرس لیلا فیروزجنگ
آدرس مطلب لیلا فیروزجنگ : درحال بروزرسانی آدرس پزشک
هزینه ویزیت لیلا فیروزجنگ چقدر است؟
برای مشاهده هزینه ویزیت دکتر و دریافت نوبت لیلا فیروزجنگ را در ابتدای همین صفحه تکمیل نمایید.
مشاوره آنلاین لیلا فیروزجنگ
از دکتر خوب براحتی میتوانید مشاوره آنلاین لیلا فیروزجنگ را دریافت کنید.
درباره لیلا فیروزجنگ
با وب سایت دکتر خوب می توانید از لیلا فیروزجنگ متخصص مامایی نوبت اینترنتی بگیرید. در صورت نیاز به ویزیت حضوری پزشک می توانید از امکان مسیریابی روی نقشه (گوگل مپ و موارد مشابه) استفاده کنید. در بسیاری از موارد ، مشاوره تلفنی با پزشک بصورت آنلاین می توانند راه حل مناسبی قبل از مراجعه حضوری به دکتر باشد که این امکان با طراحی سایت دکتر خوب امکان پذیر شده است
رشته مامایی
یکی از پرطرفدارترین زیرمجموعه های رشته های علوم پزشکی رشته مامایی است که نقش بسیار مهم و قابل توجهی در زمینههای پزشکی دارد.
نقش عمده ماما در ارتباط با مادر و کودک است.
در واقع ما به مشاوره قبل بعد و حین ازدواج و همچنین اموزش نحوه تنظیم خانواده و مراقبت از کودک در دوران بارداری و زایمان طبیعی و سزارین و همچنین مراقبت بهداشتی مادر و کودک در زمینه های بهداشت به عهده ماما می باشد.
رشته مامایی فعالیت خود را میتواند از دوران قبل از حاملگی شروع کند و حتی بعد از زایمان شش سالگی کودک نیز ادامه داشته باشد.
قبل از دوره حاملگی ماما این موضوع را بررسی می کند که آیا یک خانم توانایی باردار شدن را دارد یا خیر.
اگر باردار شدن برایش خطر داشته باشد و یا برای فرزندش مشکلی به وجود آورد باید قبل از بارداری با ماما مشورت کند و آزمایش های لازم را انجام دهد تا بارداری و زایمان بی خطری داشته باشد.
مامایی
این رشته در مقاطع کاردانی و کارشناسی دارای گرایش نیست و به صورت عمومی ارائه می شود.
ولی در مقطع کارشناسی ارشد دارای دو گرایش بهداشت مادر و کودک و گرایش آموزش مامایی می باشد.
ماما همانند رشته پزشکی و پرستاری از جمله رشتههای هست که فردی که در این رشته تحصیل میکند باید احساس مسئولیت و وظیفه و علاقه داشته باشد.
اگر علاقه و عشق و مسئولیت پذیری کافی را نداشته باشد نمی تواند پاسخگوی نیاز بیماران باشد.
ماما به فردی گفته میشود که تحصیلات مامایی را در مقطع کارشناسی و کارشناسی ارشد طبق مقررات آموزش به پایان رسانده باشد و پروانه رسمی مامایی را داشته باشد.
ماما می تواند مسئولیت نظارت مراقبت و نگهداری از زنان در دوره بارداری و زایمان و بعد از زایمان و هدایت آنها را تا زمان به دنیا اوردن فرزند و شیرخوارگی و تا 6 سالگی ادامه دهد.
ماما همچنین در مشاوره و آموزش بهداشت وظیفه مهمی را در قبال زنان جامعه انجام می دهد. کارماما آماده کردن والدین برای آشنایی با وظایفشان است.
تاریخچه مامایی
قدمت رشته مامایی در ایران به شکل آموزش عالی پس از تحصیلات دبیرستانی, به هشتاد سال قبل بر می گردد.
در سال ۱۳۱۳دانشگاه تهران تاسیس و سپس دانشکده پزشکی افتتاح گردید و در سال ۱۳۱۹بیمارستان زنان, ضمیمه دانشکده پزشکی شد.
در سال ۱۳۳۵تا ۱۳۵۸برنامه آموزشی آموزشگاه به برنامه۵/۱ساله پرستاری و مامایی برای لیسانسیه های پرستاری تغییر یافت و به دنبال سه سال تعطیلی انقلاب فرهنگی از آغاز سال ۱۳۶۲به نام “مدرسه عالی مامایی ” شروع به کار کرد.
مسئولیت ماما
ماما موظف است که گزارش را با جزئیات حفظ و نگهداری کند. گزارشی که ماما را جمع آوری می کند با گزارش که پزشک تهیه میکند جدا است.
ماما باید تمامی گزارشات تاریخچه مامایی و معاینات قبل از زایمان را انجام دهد و نگه دارد.
ماماها با بسیاری از خانواده ها و مسائل محرمانه آنها برخورد خواهند داشت و باید بسیار رازدار باشند و در برابر اطلاعاتی که از خانواده ها دارند مسئول هستند و باید بدون سرزنش و هیچ گونه کار خارج از وظیفه ای مسئولیت ماما بودن خود را انجام دهد.
ماما باید برای حفظ استانداردهای شغل و بهبود مراقبت ها همیشه فعالیت داشته باشد که لازمه این کار آگاهی داشتن از فعالیتهای سازمان های حرفه ای و هیئت های قانونی است و برای عرضه بهترین مراقبت ها باید همیشه خودش را از نظر علمی به روز نگه دارد.
سوالات متداول
به غیر از مسائل مربوط به زنان باردار، ماما وظایف دیگری نیز دارد.
این وظایف شامل مشاوره های پیش از ازدواج ، بهداشت نسل ، بهداشت زنان ، انجام معاینات و تشخیص بیماریهای زنان و راهنمایی در امر تنظیم خانواده است.
ماما ها می توانند مشاوران خوبی برای زنان در امر سبک زندگی و تغذیه مناسب در دوران پیش از بارداری باشند ، لذا میتوانید بهترین ماما بر اساس نظرات کاربران را با کلیک روی گزینه بهترین ماما تهران انتخاب کنید .
وظیفه ماما قبل از تولد نوزاد، توصیه به مادران باردار جهت داشتن تغذیه ای سالم ، دادن توضیحات لازم درمورد نحوه زایمان ، برگزاری کلاس های آموزشی برای مادران و بررسی سلامت مادر و جنین در طول بارداری است.
متخصص زنان و زایمان مشکلات و بیماریهای مربوط به زنان را تشخیص داده و درمان می کند.
پزشک زنان تستهای مختلفی را با هدف سلامت زنان انجام می دهد.
از جمله این تست ها میتوان به تست پاپ اسمیر ، STD ، آزمون سینه ، و کنترل باروری اشاره کرد.
این پزشکان هم چنین تمامی بیماری ها و مشکلات مربوط به زایمان ، بارداری ، قاعدگی ، باروری ، عفونت های مقاربتی ، اختلالات هورمونی و … را درمان میکنند.
همکاری با ما
دکتر خوب پلتفرمی است که مردم در آن متخصصان سلامت مثل روانشناسان، پزشکان و دندانپزشکان رو پیدا میکنند و از آنها اینترنتی وقت مراجعه میگیرند.
با توجه به رشد کنونی کسب و کار به همکارانی نیاز داریم که بتوانند در محیط چالشی راهحلهای خلاقانه ارائه بدهند و با توانمندی خودشان به ما در توسعه و رشد سریع پزشک خوب کمک کنند.
– فضای کار سالم و حرفهای
– همکاران حرفهای و اخلاق مدار و پرتلاش
– کار در استارتاپی که در مرحله رشد و سود دهی است
– یکی از برترین وب سایت های پزشکی
– اهمیت دادن به نظرات و خلاقیت شما
– ساعات کاری انعطاف پذیر
– حقوق ترکیبی ثابت و عملکرد محور
– بیمه تکمیلی و مزایای قانونی